Functions

by Matthew Martin
Contents
Return
Type
Parameters
Recursion
Overloading
Default
Arguments
Scope
Global
Local

top
of page
Functions are the basic unit of code in C++. Functions are provided with
a return type, a name and may have a list of parameters (arguments). The
body of the function code begins with an open curly brace and end with
a close curly brace, is indented (usually by one tab) and may contain
many lines of code.
return_type name (parameter_list)
{
body lines of code
}
top
of page

Return Type
When a function finishes executing and control is returned to the calling
function the function will return a value to the point in the code where
it was originally called from. This value must have a defined type, and
this type is defined by the return type.
The diagram below illustrates a function call to the
function myfunc, passing it the value of the variable a. The called function
receives this parameter into the local variable count. On completion of
the myfunc function the value of the variable count is returned to the
point from which myfunc was called, and this value is placed into the
variable usernumber. The thick arrows indicate the direction of code execution.
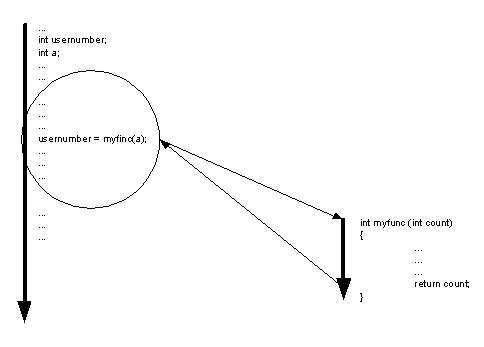
The return type may be any type available or if no value is to be returned
the keyword void is used instead and the keyword return is used at the
end of the function with no variable.
top
of page
Parameters
Parameters are values passed to the function being called. Values passed
to a function are called parameters. Once received they are called arguments.
The function has a list of parameters, where the type and name of each
is declared, separated by a comma. The types passed must be compatible
with the parameters list of the function.
If no parameters are to be passed to the function in
C++ the parameter list may be left empty . In C the keyword void must
be used.
top
of page

Recursion
Functions may call themselves: This is called recursion.
int factr (int n)
{
int answer;
if (n==1) return 1;
answer = factr (n-1) * n;
// The following line displays values to the screen.
printf ("\n answer: %i", answer);
printf ("\n n: %i", n);
printf ("\n");
return answer;
}
The best way to understand the above code is to write
a short command line (console) program. The factr() function can be called
from a main() function.
top
of page

Overloading
Functions may be overloaded by giving two or more functions the same name.
Which function is used at the time of execution will depend on the type
and number of parameters passed to the function. The compiler will sort
this out for you, so that at run time the function matching the types
and number of parameters is used (called).
top
of page

Default Arguments
In C++ (typically not in C) you can provide default values for arguments.
This means that if a function is called and no value is provided for an
argument (i.e. no parameter is passed), then the default value is used
instead.
e.g.
void myfinc(int a=0, int b = 100)
{
…
return;
}
top
of page
Scope
Scope determines the visibility and lifetime of variables and objects.
There are two main categories of scope: global and local.
Global
Global scope means that the variable is visible throughout
the program and exists for the lifetime of the program.
Local
Local scope is defined within a code block, defined by curly braces, {}.
The visibility and life time of the variable is from the beginning of
the open curly brace and ends at the closing curly brace.
A blocks of code can be nested, local scopes can be nested too. This means
that there can be more than one variable at a time with the same name
existing within differently, separately defined scopes. Some care must
be taken to avoid confusion in such cases.

by Matthew
Martin |